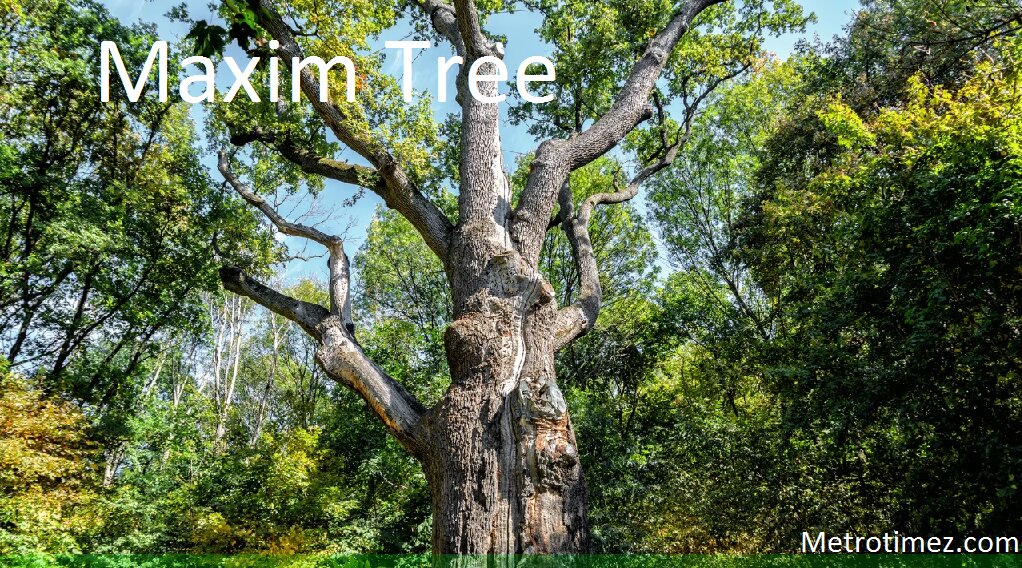
Introduction
A Maxim Tree is a specialized data structure used in computer science for storing hierarchical data in a way that allows for efficient searching, insertion, and deletion. It is designed to maintain a dynamic set of data, ensuring the maximum or minimum value of the data set is easily accessible at any time. This structure is particularly significant in scenarios where such operations are frequent, and speed is of the essence.
History of the Maxim Tree
The concept of the Maxim Tree has evolved over the years, drawing inspiration from earlier tree structures like Binary Trees and Heaps. Initially used in theoretical computer science, Maxim Trees have found practical applications in a variety of domains such as databases, networking, and artificial intelligence. Key contributors to the development of this concept include computer scientists who specialized in algorithm design and data structure optimization.
Understanding the Structure of a Maxim Tree
A Maxim Tree consists of nodes, each of which contains a data value and references to its child nodes. The tree is structured such that the value of each parent node is greater than or equal to the values of its children. This hierarchical arrangement allows for quick access to the largest value in the tree, making operations like finding the maximum efficient.
Maxim Tree Step-by-Step Instructions
To build your Maxim Tree, start by selecting a flat surface. Gather the wooden timbers and plastic parts from your set. Begin with a sturdy base, using larger pieces for stability. Gradually add layers, ensuring balance as you go. Encourage creativity by experimenting with shapes and designs—there’s no wrong way to create! Enjoy the process!
How Does a Maxim Tree Work?
At its core, a Maxim Tree functions by maintaining a heap property. When a new element is inserted, it is initially placed at the end of the tree and then “bubbled up” to its correct position by comparing it with its parent nodes and swapping where necessary. Deletion of the maximum element involves removing the root node and re-adjusting the tree to maintain the heap property.
Applications of Maxim Trees in Computer Science
Maxim Trees are widely used in various algorithms that require frequent access to the largest or smallest element. For example, they are crucial in priority queue implementations, where elements need to be processed based on priority. Additionally, Maxim Trees play a vital role in data management systems, where efficient data retrieval is necessary.
Maxim Tree vs. Other Trees
While a Maxim Tree is similar to other tree structures, such as the Binary Search Tree (BST), AVL Tree, and Red-Black Tree, it has distinct differences. Unlike a BST, which organizes nodes based on a specific ordering property, a Maxim Tree focuses on maintaining the maximum element at the root. AVL Trees and Red-Black Trees are self-balancing, while a Maxim Tree may not be balanced but maintains a partial order property.
Advantages of Using a Maxim Tree
One of the primary benefits of a Maxim Tree is its efficiency in retrieving the maximum value, which is an O(1) operation. Additionally, it provides good performance for insertion and deletion operations, typically operating in O(log n) time complexity. The structure is also less complex than some balancing trees, making it easier to implement and understand.
Limitations of a Maxim Tree
Despite its advantages, a Maxim Tree is not without limitations. It may require significant memory overhead due to its structure, particularly in large-scale applications. Additionally, in cases where balanced trees are necessary for uniformly distributed operations, Maxim Trees may not be the optimal choice.
Implementing a Maxim Tree in Programming
Here is a simple example of how to implement a Maxim Tree in Python:
python
Copy code
class MaximTree:
def __init__(self):
self.tree = []
def insert(self, value):
self.tree.append(value)
self._bubble_up(len(self.tree) – 1)
def _bubble_up(self, index):
parent_index = (index – 1) // 2
if parent_index >= 0 and self.tree[index] > self.tree[parent_index]:
self.tree[index], self.tree[parent_index] = self.tree[parent_index], self.tree[index]
self._bubble_up(parent_index)
def get_max(self):
return self.tree[0] if self.tree else None
def delete_max(self):
if len(self.tree) > 1:
max_value = self.tree[0]
self.tree[0] = self.tree.pop()
self._bubble_down(0)
elif self.tree:
max_value = self.tree.pop()
else:
max_value = None
return max_value
def _bubble_down(self, index):
largest = index
left_child = 2 * index + 1
right_child = 2 * index + 2
if left_child < len(self.tree) and self.tree[left_child] > self.tree[largest]:
largest = left_child
if right_child < len(self.tree) and self.tree[right_child] > self.tree[largest]:
largest = right_child
if largest != index:
self.tree[index], self.tree[largest] = self.tree[largest], self.tree[index]
self._bubble_down(largest)
Optimizing a Maxim Tree for Better Performance
To optimize a Maxim Tree, consider minimizing space by using efficient data structures for nodes, such as linked lists instead of arrays. Additionally, balancing insertions and deletions can help maintain a more balanced tree, reducing the overall time complexity of operations.
Real-World Scenarios Where Maxim Trees are Useful
Maxim Trees are applied in various fields like financial analysis, where priority-based data retrieval is necessary, and in networking, where data packets need to be processed in order of priority. They are also useful in implementing task scheduling systems.
Future Prospects of Maxim Trees in Technology
With the continuous evolution of technology, Maxim Trees are expected to play a more significant role in big data analytics and machine learning algorithms, where large datasets require efficient processing. Research is ongoing to improve their balancing and space optimization properties.
Common Mistakes When Working with Maxim Trees
Common errors include failing to maintain the heap property during insertion and deletion and not optimizing for space in large-scale implementations. Avoid these mistakes by thoroughly understanding the underlying mechanics and testing implementations extensively.
Tools and Resources for Learning More About Maxim Trees
Books such as “Introduction to Algorithms” by Cormen et al., websites like GeeksforGeeks, and online courses from platforms like Coursera and Udemy provide valuable insights into Maxim Trees. Additionally, forums like Stack Overflow offer community support for learners and developers.
Conclusion
Maxim Trees offer an efficient solution for storing and retrieving the maximum value in a dynamic set of data. While they have their limitations, their advantages make them an essential tool in computer science, especially in fields where speed and efficiency are paramount.
FAQs about Maxim Tree
What is a Maxim Tree?
A Maxim Tree is a specialized data structure that ensures the maximum value is always accessible at the root of the tree.
How does a Maxim Tree differ from a Binary Search Tree?
Unlike a Binary Search Tree, which organizes nodes based on a sorting order, a Maxim Tree focuses on maintaining the maximum element at the root.
What are the main applications of a Maxim Tree?
Maxim Trees are primarily used in priority queues, data management, and algorithms requiring frequent access to the largest or smallest element